Mastering Parallel Computing: A Guide to Conquer Tough Programming Assignments
Welcome to the world of parallel computing programming assignments, where the challenge lies in optimizing algorithms to harness the power of multiple processors and boost overall performance. In this blog, we'll delve into a formidable question that many students encounter at the university level. Our goal is to demystify the complexities of parallel computing while providing a step-by-step guide to tackling a challenging assignment question.
Assignment Question:
Consider a parallel computing scenario where you are tasked with implementing a parallel algorithm to find the sum of an array of integers. However, there's a twist – the algorithm should be designed to minimize communication overhead and maximize efficiency. How would you approach this task?
Understanding the Concept:
Before delving into the solution, let's grasp the fundamental concepts of parallel computing. Parallel computing involves breaking down a complex problem into smaller tasks that can be executed simultaneously by multiple processors. The key challenge is to orchestrate these parallel tasks efficiently, ensuring minimal communication overhead and optimal resource utilization.
Step-by-Step Guide:
1. Divide and Conquer:
Begin by breaking down the problem into smaller sub-problems that can be solved independently by different processors. In our case, divide the array into segments, assigning each segment to a separate processor.
2. Parallelize the Computation:
Implement the algorithm to calculate the sum of each segment in parallel. Utilize parallel programming constructs such as threads or processes to perform these computations concurrently.
3. Minimize Communication Overhead:
Since communication between processors can introduce overhead, aim to minimize it. Choose an efficient communication strategy, such as using shared memory or employing a parallel reduction algorithm to consolidate partial sums without excessive communication.
4. Optimize Load Balancing:
Ensure an even distribution of workload among processors to prevent bottlenecks. Adjust the segment sizes dynamically if necessary, based on the processing power of each processor.
5. Implement Synchronization:
Introduce synchronization mechanisms to coordinate the parallel execution of tasks. This prevents race conditions and ensures that the final result is accurate.
Sample Solution:
Here's a simplified sample solution in Python using the 'concurrent.futures' module:
import concurrent.futures
def parallel_sum(arr, num_processors):
segment_size = len(arr) // num_processors
with concurrent.futures.ThreadPoolExecutor(max_workers=num_processors) as executor:
futures = [executor.submit(sum, arr[i:i+segment_size]) for i in range(0, len(arr), segment_size)]
total_sum = sum(f.result() for f in futures)
return total_sum
This example employs Python's concurrent.futures module to implement a parallel sum algorithm, dividing the array into segments and calculating partial sums concurrently.
How We Can Help:
Navigating through complex parallel computing assignments can be challenging, and that's where our assignment help service comes in. At https://www.matlabassignmentexperts.com/parallel-computing-homework-project-help.html, we understand the difficulties students face with programming tasks. Our team of experienced programmers and educators is dedicated to providing expert parallel computing programming assignment help. Whether you need guidance, code reviews, or complete solutions, we've got you covered.
Conclusion:
Mastering parallel computing programming assignments requires a solid understanding of the underlying concepts and effective implementation strategies. By following the step-by-step guide and exploring the sample solution provided, you can approach similar challenges with confidence. Remember, if you ever find yourself in need of extra support, matlabassignmentexperts.com is here to help you conquer parallel computing assignments and excel in your academic journey.
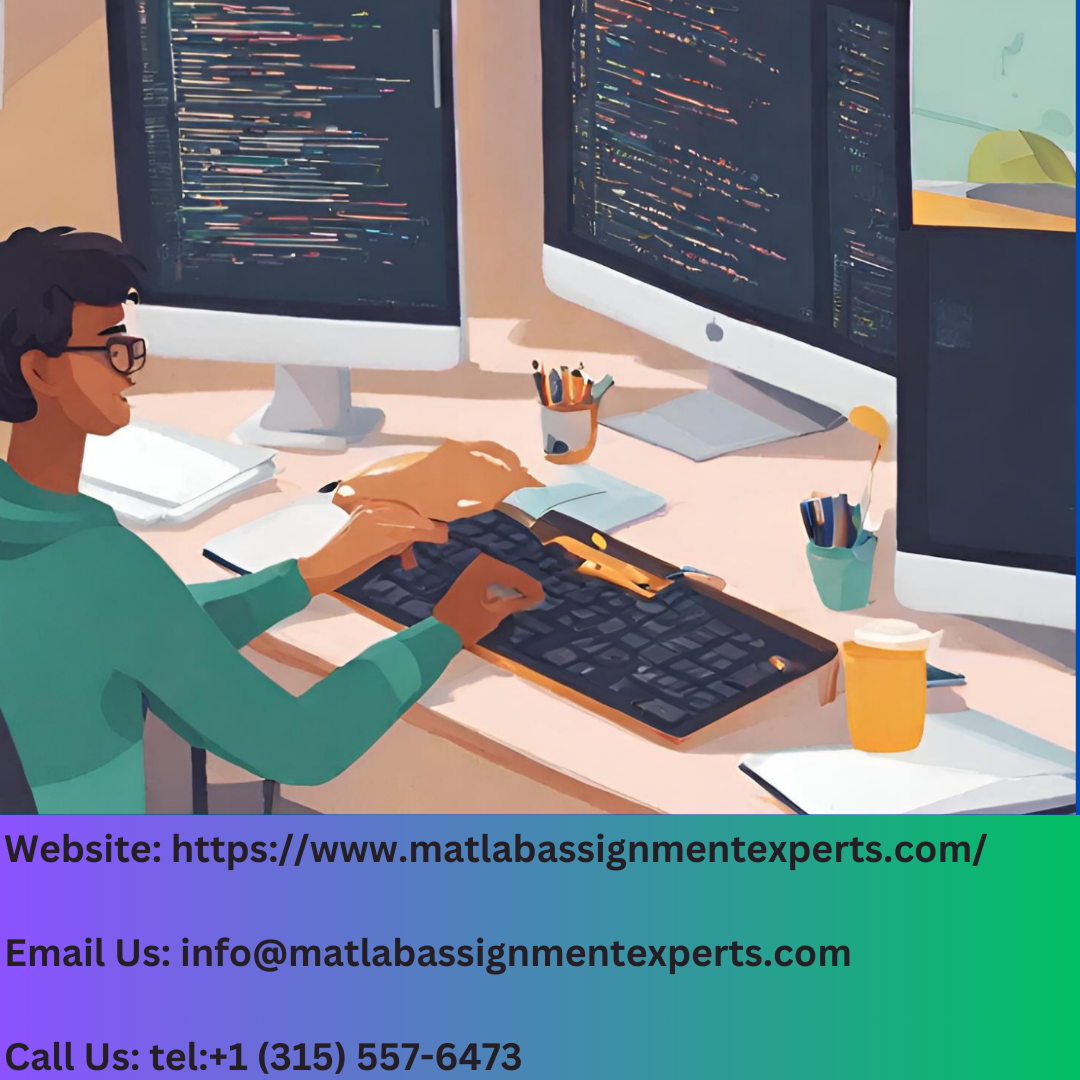